Arrays
What’s an array? #
Array is a special variable which can hold more than one value at a time.
If you have a list of items (for example viewer names), storing them in single variables would look like this:
- var name1 = “Lioran”
- var name2 = “Neverwho”
- var name3 = “Daryl”
This seems quite inefficient if you happen to have a lot of names to store. The answer is to store them all in an array, which can hold many values under a single name: names = ["Lioran", "Neverwho", "Daryl"]
.
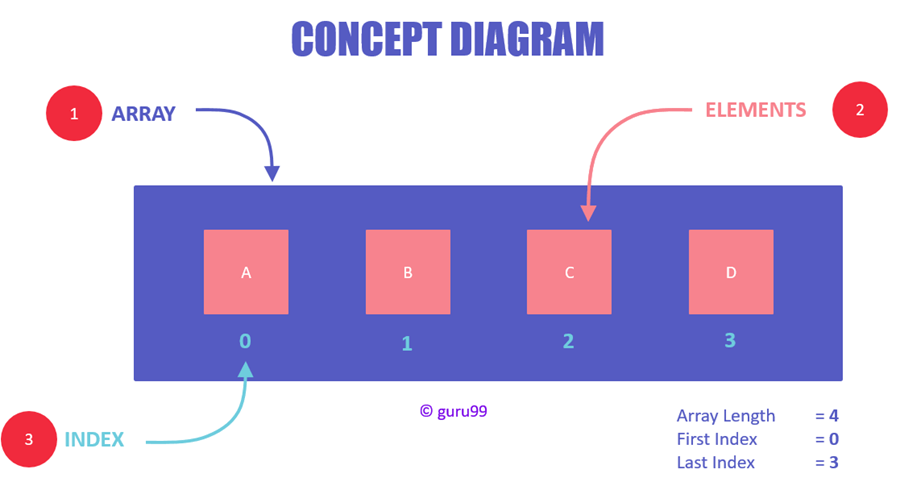
Array manipulation #
If you want to retrieve a specific value(element) from an array, you can use the following formatting:
arrayName[position]
If we have an array names = ["Lioran", "Neverwho", "Daryl"]
, typing names[1]
would retrieve 'Neverwho'
(the first value of an array has position of 0).
Array position (also called index) can contain other variables or complex math, too.
Some command boxes allow you to use not only position, but also top for retrieving and manipulating values in an array. Selecting top will target a value at the END of an array (last index). 0 will target a value at the BEGINNING of an array (index of 0).
Array is cleared every time you close or reset Receiver. If you wish to save it, you can use Array Stringify and File: Save String commands.
Convert stacks saved in .ini files from LB1 to arrays in LB2 #
If you’re using the same .ini files from LioranBoard 1, you will notice that your stringified stacks don’t get properly parsed when loaded back into LioranBoard 2 (they end up being objects instead of arrays).
Use this Stack to Array converter button:
Creates a new empty array.
You can populate it with Array Insert command.
Box Name | Type | Description |
---|---|---|
Array Name | String | Name of the new array |
Inserts a value into an array in a specific index (position), shifting other values to the right.
The position you can insert the value to can be:
-
top - adds a value to the end of the array (same as
Array push
in Javascript)Add a value to the end of the stack
-
0 - adds a value to the beginning of the array (same as
Array unshift
in Javascript)Add a value to the beginning of the stack
-
index (position) to insert the value to (similar to
Array splice
in JavaScript)Visual representation of inserting a value into an array by index
Box Name | Type | Description |
---|---|---|
Array | String | Name of the array |
Position | Position to add the value to. Can be top , 0 or index (position) of the value. | |
Value | Value you want to add to the array |
Replaces a value inside an array, overriding the previous one. Position of all values remains the same.
Pulls a value from an array by removing it from the array and saving it inside the selected variable. All remaining array value will be shifted by one.
Removes a value from an array from a specified index (position), shifting other values to the right.
This is similar to Array splice()
method in JavaScript.
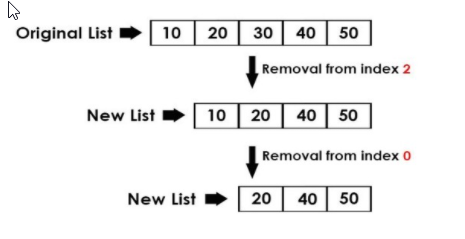
yourArrayName[valueIndex]
to remove a value, both commands will achieve the same.
Box Name | Type | Description |
---|---|---|
Array | String | Name of the array |
Delete Position | Position to delete the value at. Can be top , 0 or index (position) of the value. |
Finds the first instance of a given value inside the array and returns its index(position) in the array.
Returns -1 if value was not found.
Box Name | Type | Description |
---|---|---|
Array Name | String | Name of the array to search for the value |
Save Array Slot | String | Name of the variable to save the index(position) of the found value |
Find value | The value to find in the array |
Find value | array | Result |
---|---|---|
“blue” | [“yellow”,”red”,”blue”,”orange”,”blue”] | 2 |
“blue” | [“yellow”,”red”,”blue cat”,”orange”] | -1 (not found) |
“blue” | [“yellow”,”Blue”,”blue cat”,”blue”] | 3 |
Returns a random value inside an array.
Very useful for displaying a random image or playing a random sound.
The randomization will happen with an equal chance for each value.
_weight
added to its name and add values that will act as weights (chances to happen) to add to the randomization.
Box Name | Type | Description |
---|---|---|
Array | String | Name of the stack |
Variable (value) | String | Variable name to save the random value into |
Variable (position) | String (optional) | You can enter another variable name if you wish to get the index(position) of the random value in the array |
Sorts an array alphabetically or numerically (depending on its values) in an ascending/descending order.
If you have a mix of real and string values in your array, it will sort it based on the first array value.
Box Name | Type | Description |
---|---|---|
Array | String | Name of the array you want to sort |
Ascending | Checkbox | Checked = sort in ascending order, unchecked = sort in descending order |
Shuffles an array, randomizing the order of every value in it.
Box Name | Type | Description |
---|---|---|
Array | String | Name of the array you want to shuffle |
Returns the size of the array (= how many items it holds) and saves it into the given variable.
Returns 0 if the array is empty.
Box Name | Type | Description |
---|---|---|
Array Name | String | Name of the array |
Variable | String | Variable name to save the size of the array |
Joins two arrays together. Appends another array at the end of first array.
Both arrays must already exist.

Box Name | Type | Description |
---|---|---|
Array Name | String | The array to append to |
Array to transfer | String | The array to append at the end of the first array |
Returns a JSON string of the whole array.
You can use this command together with File: Save String command to save the whole array into a file. Once loading it back from the file, you can use Parse Array/Object command to turn the JSON string back into the original array.
Box Name | Type | Description |
---|---|---|
Variable | String | Variable to save the JSON string of the array into |
Array Name | String | Name of the array to stringify |
Turns a JSON string into an array/object. Must be properly formatted (LioranBoard will give you a warning if it finds any formatting errors).
This way you can easily create a prepopulated object/array, as it supports nesting.
Read more about JSON syntax at w3schools.com.
Box Name | Type | Description |
---|---|---|
Array/Object Name | String | Name of the variable to save the parsed array/object into |
String Array/JSON | JSON String | JSON string to parse |
JSON string before conversion | Result saved in the variable |
---|---|
["Lioran", "Melonax", "Cyanidesugar"] |
![]() |
["Hello", "Hi", {"MyObject": [1, 2, 3]}] |
|
["Lioran", "Melonax", ["cat", "rabbit", "dog"]] |
The inner array does NOT get parsed. ![]() |